Installations
npm install react-multi-date-picker
Developer
Developer Guide
Module System
CommonJS
Min. Node Version
Typescript Support
Yes
Node Version
16.20.0
NPM Version
8.19.4
Statistics
839 Stars
484 Commits
102 Forks
5 Watching
5 Branches
11 Contributors
Updated on 27 Nov 2024
Languages
JavaScript (89.58%)
CSS (10.42%)
Total Downloads
Cumulative downloads
Total Downloads
5,327,578
Last day
2.6%
14,363
Compared to previous day
Last week
4.8%
72,931
Compared to previous week
Last month
10.4%
297,509
Compared to previous month
Last year
68.4%
2,833,312
Compared to previous year
Daily Downloads
Weekly Downloads
Monthly Downloads
Yearly Downloads
Dependencies
2
Dev Dependencies
21
DatePicker
Simple React datepicker component for working with gregorian
, persian
, arabic
and indian
calendars
with the ability to select the date in single
, multiple
, range
and multiple range
modes.
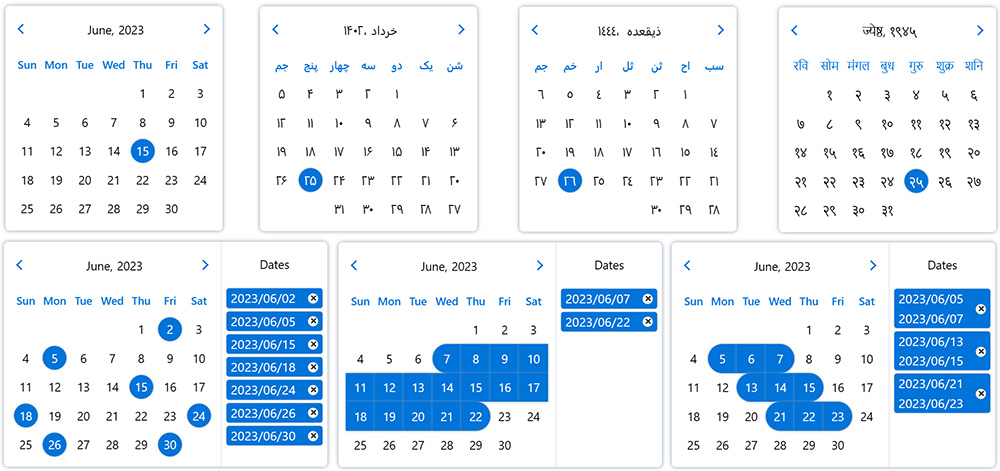
Layouts
You can change the appearance of the datepicker to prime
or mobile
by importing css files from the styles folder.

Plugins
Ability to further customize the calendar and datepicker by adding one or more plugins.
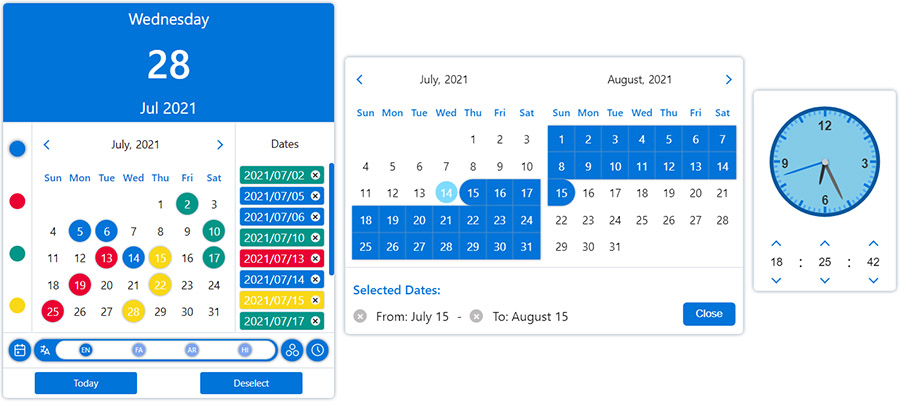
Installation
1npm i react-multi-date-picker
Demo
Usage
1import React, { useState } from "react"; 2import DatePicker from "react-multi-date-picker"; 3 4export default function Example() { 5 const [value, setValue] = useState(new Date()); 6 7 return <DatePicker value={value} onChange={setValue} />; 8}
Browser (non react-app)
1<!DOCTYPE html> 2<html> 3 <head> 4 <meta charset="UTF-8" /> 5 <title>React Multi Date Picker</title> 6 </head> 7 <body> 8 <span>Calendar Example :</span> 9 <div id="calendar"></div> 10 11 <span>DatePicker Example :</span> 12 <div id="datePicker"></div> 13 14 <span>Plugins Example :</span> 15 <div id="datePickerWithPlugin"></div> 16 17 <!-- Ract --> 18 <script src="https://unpkg.com/react@17/umd/react.production.min.js"></script> 19 <script src="https://unpkg.com/react-dom@17/umd/react-dom.production.min.js"></script> 20 21 <!-- DatePicker and dependencies--> 22 <script src="https://cdn.jsdelivr.net/npm/date-object@latest/dist/umd/date-object.min.js"></script> 23 <script src="https://cdn.jsdelivr.net/npm/react-element-popper@latest/build/browser.min.js"></script> 24 <script src="https://cdn.jsdelivr.net/npm/react-multi-date-picker@latest/build/browser.min.js"></script> 25 26 <!-- Optional Plugin --> 27 <script src="https://cdn.jsdelivr.net/npm/react-multi-date-picker@latest/build/date_picker_header.browser.js"></script> 28 29 <script> 30 const { DatePicker, Calendar } = ReactMultiDatePicker; 31 32 ReactDOM.render( 33 React.createElement(Calendar), 34 document.getElementById("calendar") 35 ); 36 37 ReactDOM.render( 38 React.createElement(DatePicker), 39 document.getElementById("datePicker") 40 ); 41 42 ReactDOM.render( 43 React.createElement(DatePicker, { 44 plugins: [React.createElement(DatePickerHeader)], 45 }), 46 document.getElementById("datePickerWithPlugin") 47 ); 48 </script> 49 </body> 50</html>
Available props
Name | Type | Default | Availability (DatePicker/ Calendar) |
---|---|---|---|
value | Date, DateObject , String, Number or Array | new Date() | both |
ref | React.RefObject | both | |
multiple | Boolean | false (true if value is Array) | both |
range | Boolean | false | both |
onlyMonthPicker | Boolean | false | both |
onlyYearPicker | Boolean | false | both |
format | String | YYYY/MM/DD | both |
formattingIgnoreList | Array | both | |
calendar | Object | gregorian | both |
locale | Object | gregorian_en | both |
mapDays | Function | both | |
onChange | Function | both | |
onPropsChange | Function | both | |
onMonthChange | Function | both | |
onYearChange | Function | both | |
onFocusedDateChange | Function | both | |
digits | Array | both | |
weekDays | Array | both | |
months | Array | both | |
showOtherDays | Boolean | false | both |
minDate | Date, DateObject, String or Number | both | |
maxDate | Date, DateObject, String or Number | both | |
disableYearPicker | Boolean | false | both |
disableMonthPicker | Boolean | false | both |
disableDayPicker | Boolean | false | both |
zIndex | Number | 100 | both |
plugins | Array | [] | both |
sort | Boolean | false | both |
numberOfMonths | Number | 1 | both |
currentDate | DateObject | both | |
buttons | Boolean | true | both |
renderButton | React.ReactElement or Function | both | |
weekStartDayIndex | Number | both | |
className | String | both | |
readOnly | Boolean | false | both |
disabled | Boolean | false | both |
hideMonth | Boolean | false | both |
hideYear | Boolean | false | both |
hideWeekDays | Boolean | false | both |
shadow | Boolean | true | both |
fullYear | Boolean | false | both |
displayWeekNumbers | Boolean | false | both |
weekNumber | String | both | |
weekPicker | Boolean | false | both |
rangeHover | Boolean | false | both |
monthYearSeparator | String | "," for LTR locales, "،" for RTL locales | both |
formatMonth | Function | undefined | both |
formatYear | Function | undefined | both |
highlightToday | Boolean | true | both |
style | React.CSSProperties | {} | both |
headerOrder | Array | ["LEFT_BUTTON", "MONTH_YEAR", "RIGHT_BUTTON"] | both |
onOpen | Function | DatePicker | |
onClose | Function | DatePicker | |
onPositionChange | Function | DatePicker | |
containerClassName | String | DatePicker | |
arrowClassName | String | 0 | DatePicker |
containerStyle | React.CSSProperties | DatePicker | |
arrowStyle | React.CSSProperties | 0 | DatePicker |
arrow | Boolean or React.ReactElement | true | DatePicker |
animations | Array | false | DatePicker |
inputClass | String | DatePicker | |
name | String | DatePicker | |
id | String | DatePicker | |
title | String | DatePicker | |
required | Boolean | DatePicker | |
placeholder | String | DatePicker | |
render | React.ReactElement or Function | DatePicker | |
inputMode | String | DatePicker | |
scrollSensitive | Boolean | true | DatePicker |
hideOnScroll | Boolean | false | DatePicker |
calendarPosition | String | "bottom-left" | DatePicker |
editable | Boolean | true | DatePicker |
onlyShowInRangeDates | Boolean | true | DatePicker |
fixMainPosition | Boolean | false | DatePicker |
fixRelativePosition | Boolean | false | DatePicker |
offsetY | Number | 0 | DatePicker |
offsetX | Number | 0 | DatePicker |
mobileLabels | Object | DatePicker | |
portal | Boolean | DatePicker | |
portalTarget | HTMLElement | DatePicker | |
onOpenPickNewDate | Boolean | true | DatePicker |
mobileButtons | HTMLButtonElement[] | [] | DatePicker |
dateSeparator | String | '~' in range mode, ',' in multiple mode | DatePicker |
multipleRangeSeparator | String | ',' | DatePicker |
typingTimeout | String | 700 | DatePicker |
autoFocus | Boolean | false | Calendar |
Calendars & Locales
Click here to see the descriptions.
Calendars | Gregorian | Persian (Solar Hijri) | Jalali | Arabic (Lunar Hijri) | Indian | |
---|---|---|---|---|---|---|
/calendars/gregorian | /calendars/persian | /calendars/jalali | /calendars/arabic | /calendars/indian | ||
Locales | English | /locales/gregorian_en | /locales/persian_en | /locales/persian_en | /locales/arabic_en | /locales/indian_en |
Portuguese - BRAZIL | /locales/gregorian_pt_br | - | - | - | - | |
Farsi | /locales/gregorian_fa | /locales/persian_fa | /locales/persian_fa | /locales/arabic_fa | /locales/indian_fa | |
Arabic | /locales/gregorian_ar | /locales/persian_ar | /locales/persian_ar | /locales/arabic_ar | /locales/indian_ar | |
Hindi | /locales/gregorian_hi | /locales/persian_hi | /locales/persian_hi | /locales/arabic_hi | /locales/indian_hi |
Of course, you can customize the names of the months and days of the week
in both the calendar & input by using the months
and weekDays
Props.
Also, you can create a custom Calendar and Locale:

No vulnerabilities found.
Reason
no dangerous workflow patterns detected
Reason
no binaries found in the repo
Reason
license file detected
Details
- Info: project has a license file: LICENSE:0
- Info: FSF or OSI recognized license: MIT License: LICENSE:0
Reason
SAST tool detected but not run on all commits
Details
- Info: SAST configuration detected: CodeQL
- Warn: 0 commits out of 5 are checked with a SAST tool
Reason
2 commit(s) and 1 issue activity found in the last 90 days -- score normalized to 2
Reason
Found 2/27 approved changesets -- score normalized to 0
Reason
detected GitHub workflow tokens with excessive permissions
Details
- Warn: no topLevel permission defined: .github/workflows/codeql-analysis.yml:1
- Info: no jobLevel write permissions found
Reason
dependency not pinned by hash detected -- score normalized to 0
Details
- Warn: GitHub-owned GitHubAction not pinned by hash: .github/workflows/codeql-analysis.yml:33: update your workflow using https://app.stepsecurity.io/secureworkflow/shahabyazdi/react-multi-date-picker/codeql-analysis.yml/master?enable=pin
- Warn: GitHub-owned GitHubAction not pinned by hash: .github/workflows/codeql-analysis.yml:46: update your workflow using https://app.stepsecurity.io/secureworkflow/shahabyazdi/react-multi-date-picker/codeql-analysis.yml/master?enable=pin
- Warn: GitHub-owned GitHubAction not pinned by hash: .github/workflows/codeql-analysis.yml:57: update your workflow using https://app.stepsecurity.io/secureworkflow/shahabyazdi/react-multi-date-picker/codeql-analysis.yml/master?enable=pin
- Warn: GitHub-owned GitHubAction not pinned by hash: .github/workflows/codeql-analysis.yml:71: update your workflow using https://app.stepsecurity.io/secureworkflow/shahabyazdi/react-multi-date-picker/codeql-analysis.yml/master?enable=pin
- Info: 0 out of 4 GitHub-owned GitHubAction dependencies pinned
Reason
no effort to earn an OpenSSF best practices badge detected
Reason
security policy file not detected
Details
- Warn: no security policy file detected
- Warn: no security file to analyze
- Warn: no security file to analyze
- Warn: no security file to analyze
Reason
project is not fuzzed
Details
- Warn: no fuzzer integrations found
Reason
branch protection not enabled on development/release branches
Details
- Warn: branch protection not enabled for branch 'master'
Reason
98 existing vulnerabilities detected
Details
- Warn: Project is vulnerable to: GHSA-prr3-c3m5-p7q2
- Warn: Project is vulnerable to: GHSA-grv7-fg5c-xmjg
- Warn: Project is vulnerable to: GHSA-3xgq-45jj-v275
- Warn: Project is vulnerable to: GHSA-952p-6rrq-rcjv
- Warn: Project is vulnerable to: GHSA-7fh5-64p2-3v2j
- Warn: Project is vulnerable to: GHSA-gcx4-mw62-g8wm
- Warn: Project is vulnerable to: GHSA-3h5v-q93c-6h6q
- Warn: Project is vulnerable to: GHSA-67hx-6x53-jw92
- Warn: Project is vulnerable to: GHSA-c2jc-4fpr-4vhg
- Warn: Project is vulnerable to: GHSA-whgm-jr23-g3j9
- Warn: Project is vulnerable to: GHSA-93q8-gq69-wqmw
- Warn: Project is vulnerable to: GHSA-fwr7-v2mv-hh25
- Warn: Project is vulnerable to: GHSA-cph5-m8f7-6c5x
- Warn: Project is vulnerable to: GHSA-wf5p-g6vw-rhxx
- Warn: Project is vulnerable to: GHSA-qwcr-r2fm-qrc7
- Warn: Project is vulnerable to: GHSA-w8qv-6jwh-64r5
- Warn: Project is vulnerable to: GHSA-pxg6-pf52-xh8x
- Warn: Project is vulnerable to: GHSA-7gc6-qh9x-w6h8
- Warn: Project is vulnerable to: GHSA-w573-4hg7-7wgq
- Warn: Project is vulnerable to: GHSA-fp36-299x-pwmw
- Warn: Project is vulnerable to: GHSA-273r-mgr4-v34f
- Warn: Project is vulnerable to: GHSA-r7qp-cfhv-p84w
- Warn: Project is vulnerable to: GHSA-4gmj-3p3h-gm8h
- Warn: Project is vulnerable to: GHSA-6h5x-7c5m-7cr7
- Warn: Project is vulnerable to: GHSA-rv95-896h-c2vc
- Warn: Project is vulnerable to: GHSA-qw6h-vgh9-j6wx
- Warn: Project is vulnerable to: GHSA-mhxj-85r3-2x55
- Warn: Project is vulnerable to: GHSA-74fj-2j2h-c42q
- Warn: Project is vulnerable to: GHSA-pw2r-vq6v-hr8c
- Warn: Project is vulnerable to: GHSA-jchw-25xp-jwwc
- Warn: Project is vulnerable to: GHSA-cxjh-pqwp-8mfp
- Warn: Project is vulnerable to: GHSA-c6f8-8r25-c4gc
- Warn: Project is vulnerable to: GHSA-8mmm-9v2q-x3f9
- Warn: Project is vulnerable to: GHSA-pfrx-2q88-qq97
- Warn: Project is vulnerable to: GHSA-rc47-6667-2j5j
- Warn: Project is vulnerable to: GHSA-c7qv-q95q-8v27
- Warn: Project is vulnerable to: GHSA-33f9-j839-rf8h
- Warn: Project is vulnerable to: GHSA-c36v-fmgq-m8hx
- Warn: Project is vulnerable to: GHSA-78xj-cgh5-2h22
- Warn: Project is vulnerable to: GHSA-2p57-rm9w-gvfp
- Warn: Project is vulnerable to: GHSA-9c47-m6qq-7p4h
- Warn: Project is vulnerable to: GHSA-76p3-8jx3-jpfq
- Warn: Project is vulnerable to: GHSA-3rfm-jhwj-7488
- Warn: Project is vulnerable to: GHSA-hhq3-ff78-jv3g
- Warn: Project is vulnerable to: GHSA-f8q6-p94x-37v3
- Warn: Project is vulnerable to: GHSA-xvch-5gv4-984h
- Warn: Project is vulnerable to: GHSA-8hfj-j24r-96c4
- Warn: Project is vulnerable to: GHSA-wc69-rhjr-hc9g
- Warn: Project is vulnerable to: GHSA-qrpm-p2h7-hrv2
- Warn: Project is vulnerable to: GHSA-r683-j2x4-v87g
- Warn: Project is vulnerable to: GHSA-5rrq-pxf6-6jx5
- Warn: Project is vulnerable to: GHSA-8fr3-hfg3-gpgp
- Warn: Project is vulnerable to: GHSA-gf8q-jrpm-jvxq
- Warn: Project is vulnerable to: GHSA-2r2c-g63r-vccr
- Warn: Project is vulnerable to: GHSA-cfm4-qjh2-4765
- Warn: Project is vulnerable to: GHSA-x4jg-mjrx-434g
- Warn: Project is vulnerable to: GHSA-rp65-9cf3-cjxr
- Warn: Project is vulnerable to: GHSA-v39p-96qg-c8rf
- Warn: Project is vulnerable to: GHSA-8v63-cqqc-6r2c
- Warn: Project is vulnerable to: GHSA-3j8f-xvm3-ffx4
- Warn: Project is vulnerable to: GHSA-4p35-cfcx-8653
- Warn: Project is vulnerable to: GHSA-7f3x-x4pr-wqhj
- Warn: Project is vulnerable to: GHSA-jpp7-7chh-cf67
- Warn: Project is vulnerable to: GHSA-q6wq-5p59-983w
- Warn: Project is vulnerable to: GHSA-j9fq-vwqv-2fm2
- Warn: Project is vulnerable to: GHSA-pqw5-jmp5-px4v
- Warn: Project is vulnerable to: GHSA-9wv6-86v2-598j
- Warn: Project is vulnerable to: GHSA-hwj9-h5mp-3pm3
- Warn: Project is vulnerable to: GHSA-566m-qj78-rww5
- Warn: Project is vulnerable to: GHSA-hqhp-5p83-hx96
- Warn: Project is vulnerable to: GHSA-3949-f494-cm99
- Warn: Project is vulnerable to: GHSA-hrpp-h998-j3pp
- Warn: Project is vulnerable to: GHSA-c2qf-rxjj-qqgw
- Warn: Project is vulnerable to: GHSA-m6fv-jmcg-4jfg
- Warn: Project is vulnerable to: GHSA-cm22-4g7w-348p
- Warn: Project is vulnerable to: GHSA-gp95-ppv5-3jc5
- Warn: Project is vulnerable to: GHSA-54xq-cgqr-rpm3
- Warn: Project is vulnerable to: GHSA-g4rg-993r-mgx7
- Warn: Project is vulnerable to: GHSA-wpg7-2c88-r8xv
- Warn: Project is vulnerable to: GHSA-25hc-qcg6-38wj
- Warn: Project is vulnerable to: GHSA-qm95-pgcg-qqfq
- Warn: Project is vulnerable to: GHSA-cqmj-92xf-r6r9
- Warn: Project is vulnerable to: GHSA-5955-9wpr-37jh
- Warn: Project is vulnerable to: GHSA-qq89-hq3f-393p
- Warn: Project is vulnerable to: GHSA-f5x3-32g6-xq36
- Warn: Project is vulnerable to: GHSA-4wf5-vphf-c2xc
- Warn: Project is vulnerable to: GHSA-w5p7-h5w8-2hfq
- Warn: Project is vulnerable to: GHSA-hh27-ffr2-f2jc
- Warn: Project is vulnerable to: GHSA-rqff-837h-mm52
- Warn: Project is vulnerable to: GHSA-8v38-pw62-9cw2
- Warn: Project is vulnerable to: GHSA-hgjh-723h-mx2j
- Warn: Project is vulnerable to: GHSA-jf5r-8hm2-f872
- Warn: Project is vulnerable to: GHSA-hc6q-2mpp-qw7j
- Warn: Project is vulnerable to: GHSA-4vvj-4cpr-p986
- Warn: Project is vulnerable to: GHSA-wr3j-pwj9-hqq6
- Warn: Project is vulnerable to: GHSA-j8xg-fqg3-53r7
- Warn: Project is vulnerable to: GHSA-6fc8-4gx4-v693
- Warn: Project is vulnerable to: GHSA-776f-qx25-q3cc
Score
3.1
/10
Last Scanned on 2024-11-18
The Open Source Security Foundation is a cross-industry collaboration to improve the security of open source software (OSS). The Scorecard provides security health metrics for open source projects.
Learn More